How to Create a Chat App in Android
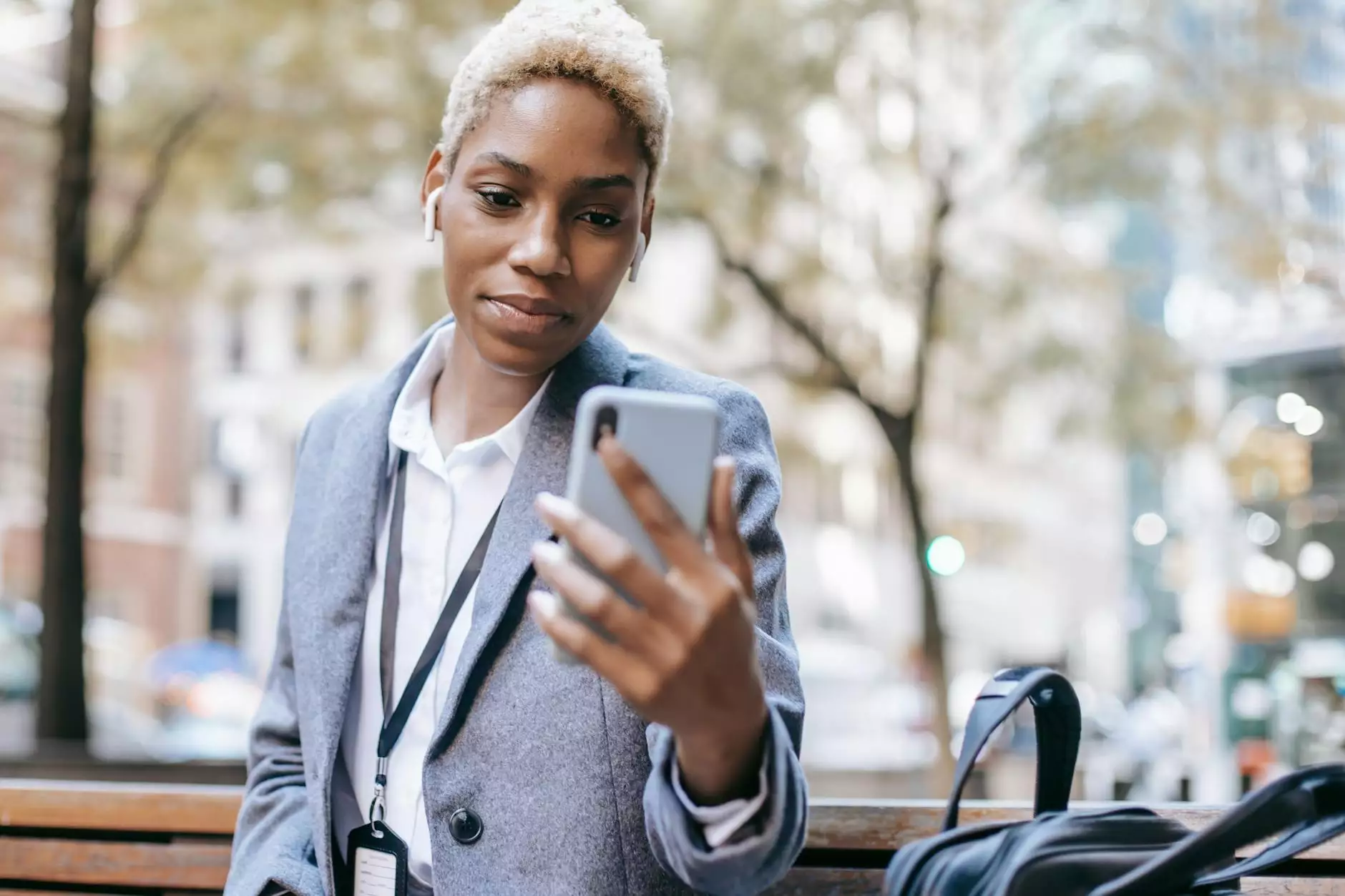
In today's digital age, communication is key, and one of the most popular ways to stay connected is through chat applications. If you're interested in learning how to create a chat app in Android, you've come to the right place. This article will provide you with a thorough guide to help you develop your own chat application seamlessly.
Understanding the Basics of Chat Applications
Before diving into the developmental aspects, it's crucial to understand what a chat application entails. Chat applications, often referred to as instant messaging apps, allow users to exchange messages in real-time. They may also include features like:
- Text Messaging: The core functionality where users can send and receive messages.
- Multimedia Sharing: Users can share images, videos, and audio clips.
- Voice and Video Calls: Some chat apps offer the ability to conduct voice and video conversations.
- User Presence: Indicators showing whether a user is online, offline, or inactive.
Moreover, features like group chats, message notifications, and end-to-end encryption can significantly enhance user experience and privacy.
Getting Started with Android Development
To develop an Android application, you need to have the following prerequisites covered:
- Android Studio: The official Integrated Development Environment (IDE) for Android development.
- Java or Kotlin Knowledge: Proficiency in either of these programming languages is essential for writing the application code.
- Firebase Account: Firebase is widely used for backend services like real-time database, authentication, and cloud messaging.
Ensure you have the latest version of Android Studio installed and create a new project with an empty activity.
Step-by-Step Guide on How to Create a Chat App in Android
Step 1: Setting Up the Project
When launching Android Studio, create a new project by selecting “Empty Activity.” Configure your project by naming it (e.g., ChatApp) and setting the desired package name. Make sure to select the appropriate API level. You can start with API level 21 (Lollipop) as it covers about 94.1% of devices.
Step 2: Adding Dependencies
Open the build.gradle file (Module: app) and add the necessary dependencies for Firebase and any libraries you plan to use, such as:
implementation 'com.google.firebase:firebase-database:20.0.0' implementation 'com.google.firebase:firebase-auth:21.0.1' implementation 'com.squareup.retrofit2:retrofit:2.9.0'Sync the project to ensure all dependencies are downloaded and configured correctly.
Step 3: Setting Up Firebase
Navigate to the Firebase Console and create a new project. Once created, add an Android app to your project. Follow the prompts to download the google-services.json file and place it in the app directory of your Android project. Add Firebase authentication and database services.
Step 4: Creating the User Interface (UI)
Designing your app’s User Interface is crucial. Utilize Android Studio’s XML layout files to create the necessary UI elements:
- Main Activity: Contains a RecyclerView to display chat messages.
- Chat Activity: A layout with EditText for message input and Send button.
Implement the necessary adapter classes to bind data to your RecyclerView for displaying messages.
Step 5: Implementing Firebase Authentication
To manage users, integrate Firebase Authentication. Use the following code snippet for email and password sign-up:
FirebaseAuth auth = FirebaseAuth.getInstance(); auth.createUserWithEmailAndPassword(email, password) .addOnCompleteListener(this, task -> { if (task.isSuccessful()) { // Sign-up successful } else { // Sign-up failed } });Once authenticated, users can send and receive messages.
Step 6: Configuring the Real-Time Database
Set up Firebase Database rules for read and write access. Ensure your database structure is organized to handle chat data efficiently. For instance:
{ "messages": { "messageId1": { "senderId": "user1", "text": "Hello!", "timestamp": "time" }, "messageId2": { "senderId": "user2", "text": "Hi!", "timestamp": "time" } } }Use Firebase's real-time capabilities to listen for new messages in the chat.
Step 7: Sending and Retrieving Messages
Implement methods for sending messages to the real-time database and updating the RecyclerView accordingly. Here’s a brief example:
DatabaseReference chatRef = FirebaseDatabase.getInstance().getReference("messages"); chatRef.push().setValue(new Message(senderId, messageText));Step 8: Testing and Debugging Your App
Before releasing your app, it is essential to conduct thorough testing. Test all functionalities, including sending and receiving messages, and check for any bugs or errors. Utilize Android Studio's debugging tools and logcat to identify issues and ensure a smooth user experience.
Advanced Features to Consider
Once you’ve successfully implemented the core functionalities, consider enhancing your chat app with advanced features such as:
- User Profile Management: Allow users to create profiles with profile pictures and status messages.
- Push Notifications: Implement Firebase Cloud Messaging (FCM) to notify users about new messages.
- Group Chats: Provide functionality for users to create and join group chats.
- Message Encryption: Enhance security by implementing end-to-end encryption for user messages.
Conclusion
In summary, learning how to create a chat app in Android is a remarkable journey that combines several essential skills in mobile development. By following this guide, you can develop a robust chat application that not only meets user needs but also stands out in the competitive app market. With attention to user interface, performance, and security, your chat app can provide a rich and interactive experience for users. As technology continues to evolve, keep learning and exploring new features to integrate into your application.
Start building your app today, and join the ever-growing community of mobile app developers who are redefining how we communicate.